Git Tutorial
Git commands are essential for version control, a system that tracks changes to your code or files over time. Here’s why they’re important:
- Track Changes: Git lets you see exactly what has changed in your project at any point in time. This is crucial for debugging issues, reverting to previous versions if needed, and collaborating effectively.
- Collaboration: Git enables multiple people to work on the same project simultaneously. It helps avoid conflicts and keeps everyone on the same page by managing different versions and merging changes.
- Backup and Restore: Git acts as a safe haven for your project’s history. If you accidentally delete something or mess up your code, you can easily restore it from a previous commit.
- Remote Repositories: You can share your project with others or store it on a remote server using platforms like GitHub. This allows for collaboration across teams and locations, and provides a secure backup solution.
- Project Management: Git commands help you organize your project into different branches, making it easier to experiment with features or bug fixes without affecting the main codebase.
Overall, Git commands are the backbone of modern software development. They streamline collaboration, ensure project safety, and provide valuable insights into your project’s evolution.
Getting Setup with Git:
Here’s how to set up your Git username and email globally with commands:
1. Checking Git Version (and Installation):
The commands git version
and git v
both achieve the same result: checking if Git is already installed.
- If Git is not installed, running these commands will typically prompt you through Xcode installation (since Xcode includes Git on macOS). Select “Yes” to install Git.
- Recommended Method: The instructions suggest using Homebrew, a popular package manager for macOS, for Git installation. Here’s how to install Git with Homebrew:
- Visit https://brew.sh/ and follow the instructions to install Homebrew if you don’t have it already.
- Open a terminal window and run the command
brew install git
to install Git using Homebrew.
2. Checking Git Configuration:
The command git config --global --list
displays your current global Git configuration settings. This is optional but helpful to see what’s already set.
3. Setting Username and Email:
git config --global user.name "My Name"
– Replace"My Name"
with your actual name. Double quotes are only needed if your name has spaces.git config --global user.email "[email protected]"
– Replace"[email protected]"
with your actual email address.
4. Setting Editor (VS Code):
Before setting the editor, you need to verify if VS Code is recognized by the terminal:
- Open a terminal window and run
code --version
. If the version information shows up, VS Code is recognized and you can proceed. - If
code --version
doesn’t work, you might need to adjust your system PATH environment variable to include the directory where VS Code is installed. Refer to the VS Code documentation for Mac setup (https://code.visualstudio.com/docs/setup/mac) for instructions on setting the PATH.
Once you’ve confirmed VS Code recognition:
- Run the command
git config --global core.editor "code --wait"
. This sets VS Code as your default editor for Git commands that require editing files (like commit messages).
5. Viewing Git Configuration Details:
git config --global -e
opens your global Git configuration file (usually.gitconfig
) in your default text editor. This allows you to view all your current settings and potentially make further customizations.
6. Configuring Line Endings (Optional):
git config --global core.autocrlf input
– This setting is specifically useful when working between Windows and Mac machines. It tells Git to handle line ending conversions automatically based on the system you’re using. You can refer to the Git documentation for more details oncore.autocrlf
(https://docs.github.com/en/get-started/getting-started-with-git/configuring-git-to-handle-line-endings).
By following these steps, you’ll have Git set up and configured on your Mac, including your username, email, preferred editor (VS Code), and optional line ending configuration.
Here are the most common Git Commands:
Here’s the reorganized list of Git commands without duplicates and grouped by functionality:
1. Repository Setup and Initialization:
git init
: Creates a new Git repository in the current directory.git clone <url>
: Clones an existing Git repository from a remote location (like GitHub) to your local machine.
2. Working with Changes:
git add <filename>
: Adds a specific file to the staging area for the next commit.git add .
: Adds all tracked files (files already added to the repository) in the current directory to the staging area.git rm <filename>
: Removes a file from the working directory and the staging area (if it’s already staged).git rm --cached <filename>
: Removes a file only from the staging area (if it’s already staged).git mv <source> <destination>
: Renames a file and updates the Git index.git checkout -- <filename>
: Discards all changes made to a file in the working directory.
3. Commits:
git commit -m "<commit message>"
: Creates a snapshot of the changes in the staging area and stores it in the Git history, along with a descriptive commit message.git commit --amend
: Amends the most recent commit by allowing you to edit the commit message.
4. Viewing Changes:
git status
: Shows the current status of your working directory, including any unstaged or staged changes.git log
: Displays the commit history of your repository.git diff
: Shows the difference between the working directory and the index, or between the index and the HEAD commit.git show <commit-hash>
: Displays details of a specific commit, including the commit message, author, and date.
5. Branching and Merging:
git branch
: Lists all branches in your local repository.git branch <branch-name>
: Creates a new branch.git checkout <branch-name>
: Switches to a different branch.git merge <branch-name>
: Merges another branch into the current branch.git rebase <branch-name>
: Rebases the current branch on top of another branch, rewriting Git history (use with caution).
6. Remote Repositories:
git remote add <name> <url>
: Adds a remote repository to your local repository (usually named “origin”).git push <remote-name> <branch-name>
: Pushes your local commits to a remote repository branch.git pull <remote-name> <branch-name>
: Pulls down changes from a remote repository branch and merges them with your local branch.git fetch <remote-name>
: Downloads changes from a remote repository without merging them into your local branch.
7. Undoing Changes:
git reset HEAD <filename>
: Unstages a file from the staging area. (Carefully consider usinggit reset
as it rewrites history)git reset --hard HEAD
: Discards all local changes made to tracked files since the latest commit. (Use with extreme caution)
8. Tags:
git tag <tag-name> <commit-hash>
: Creates a tag that references a specific commit.
9. Stashing:
git stash
: Temporarily saves your local changes without committing them.git stash pop
: Applies the most recent stashed changes to your working directory.
These are just some of the most common Git commands. As you become more comfortable with Git, you can explore more advanced features like submodules, hooks, and ignoring specific files or patterns with .gitignore
.
For a very comprehensive list with detailed explanations, you can refer to the official Git documentation: https://git-scm.com/.
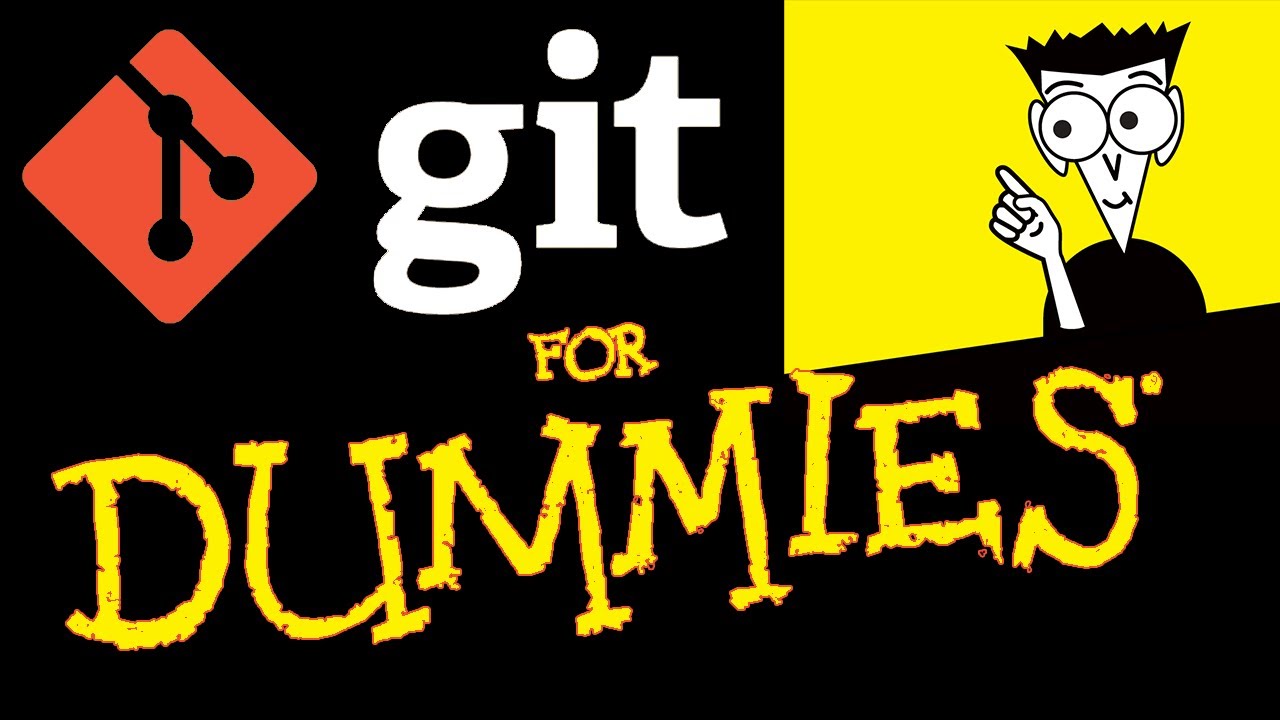
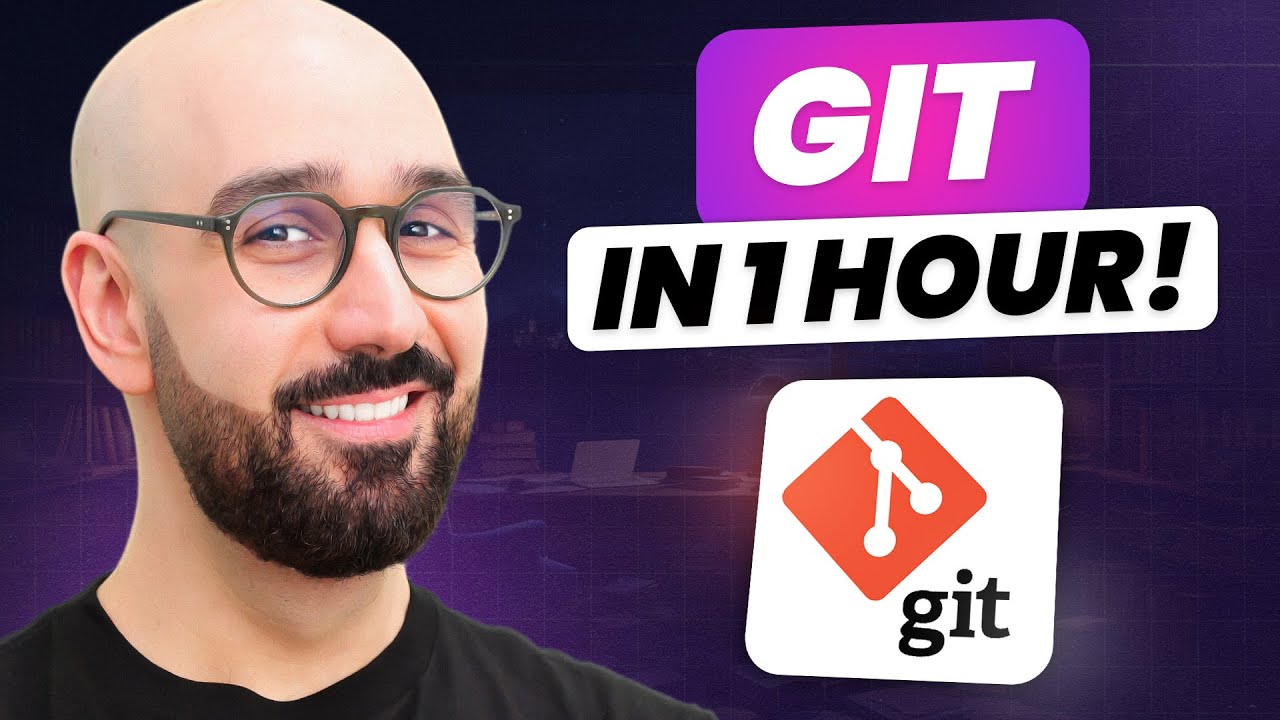
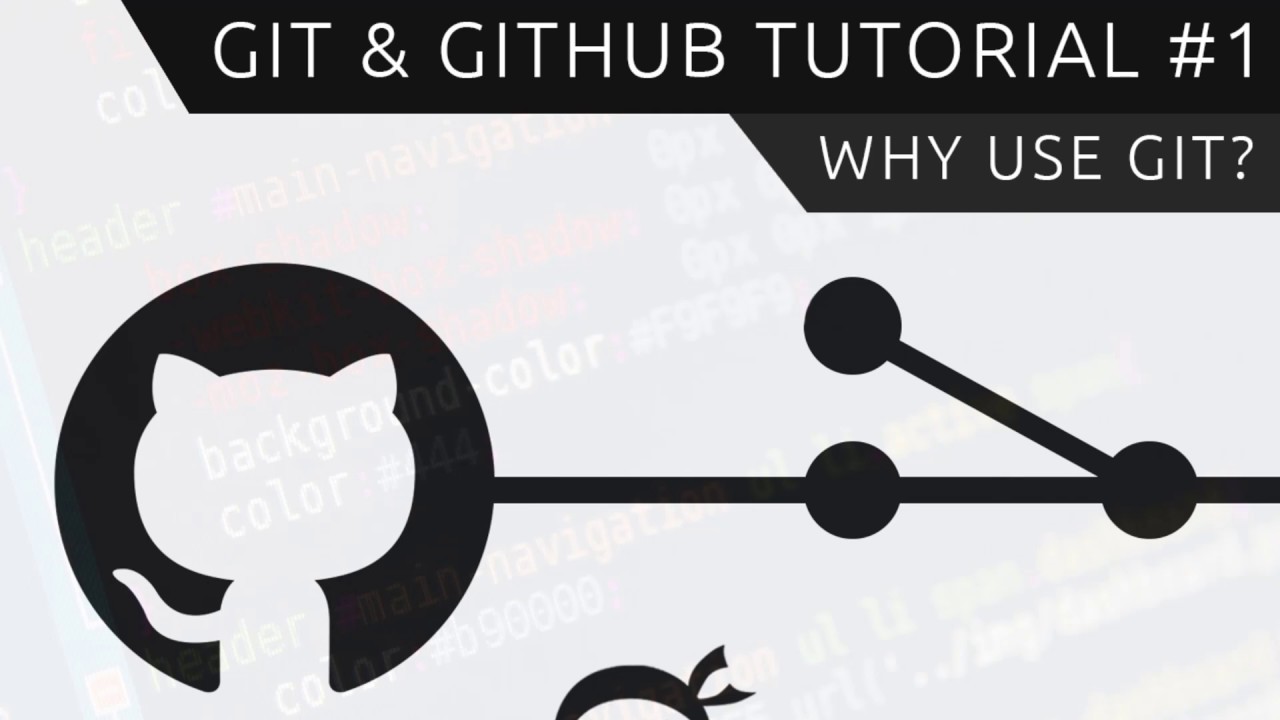
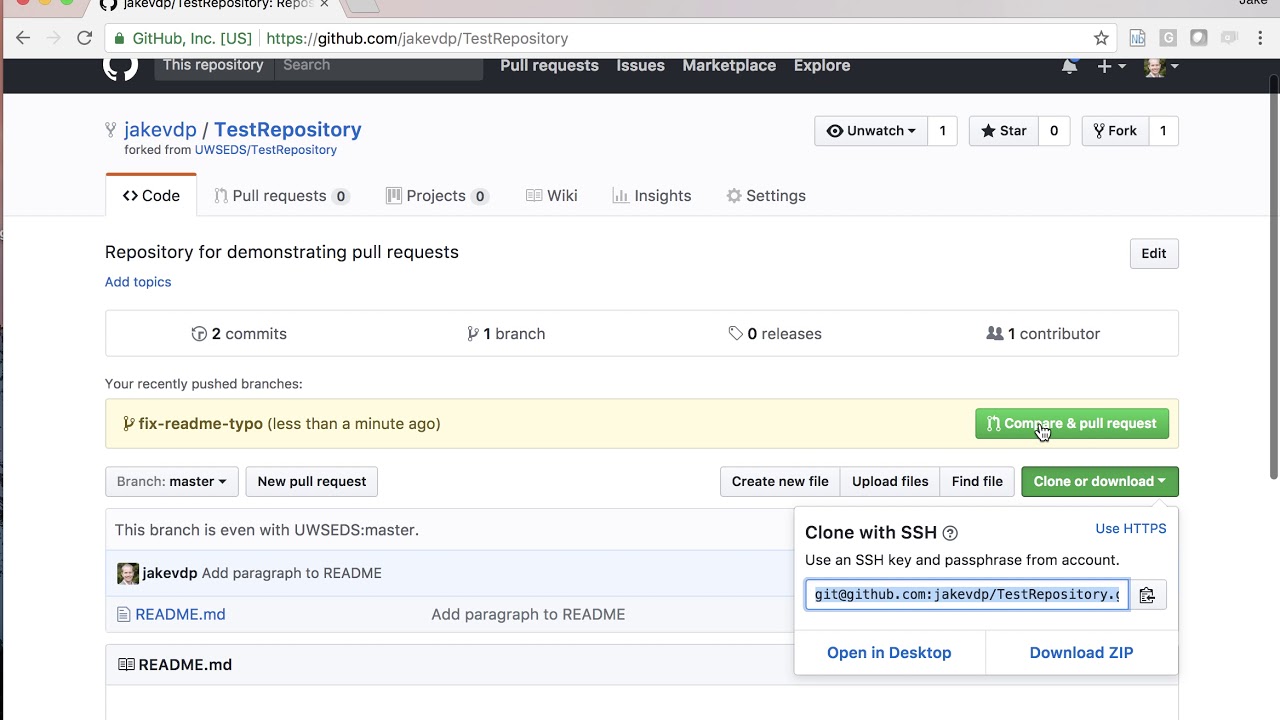