Visual Studio Code Tutorial
Visual Studio Code, VS Code for short, is a popular source code editor created by Microsoft. VS Code’s blend of lightweight performance, rich extensibility, and cross-platform compatibility make it the go-to editor for many developers. VS Code offers features like syntax highlighting, code completion, and debugging for a variety of programming languages. It’s also extremely customizable, with themes and extensions to fit your coding style and needs. Available for Windows, macOS, Linux, and even the web, VS Code is a great choice for programmers of all levels.
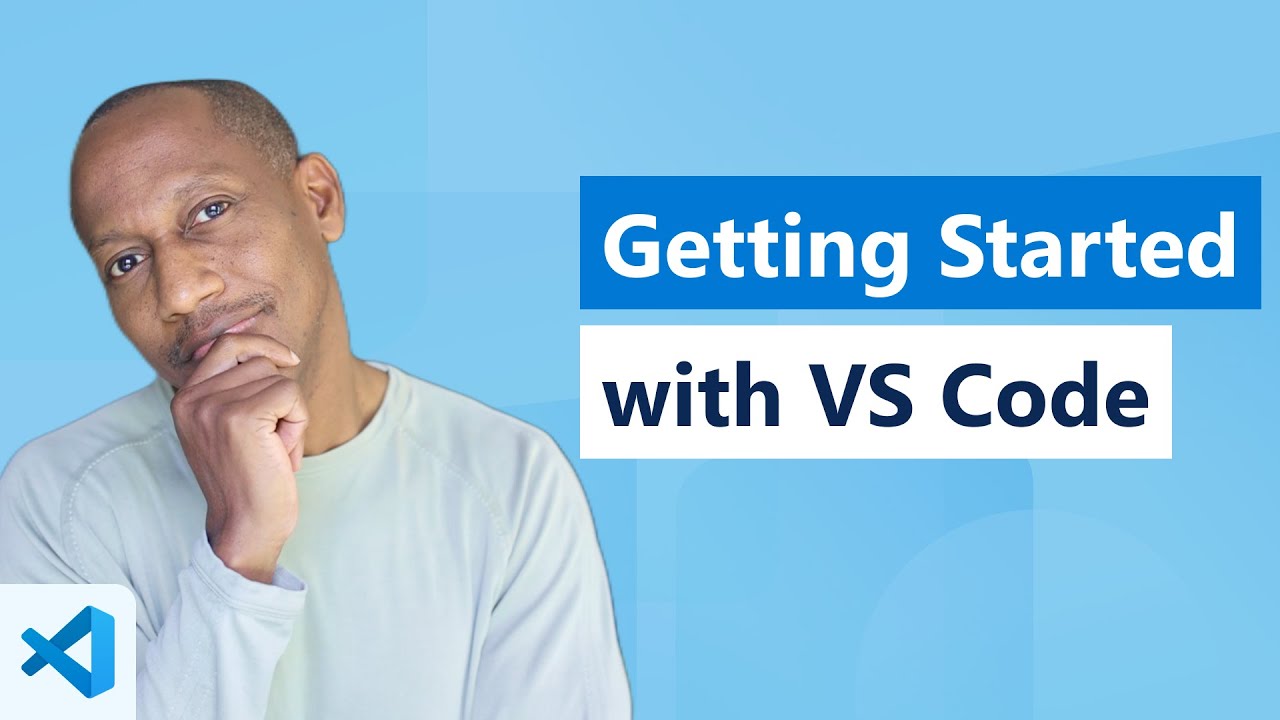
Visual Studio Code Extensions To Consider:
Essential Extensions:
- Live Server: Launches a local development server with live reloading, allowing you to see changes in your browser instantly without manual refreshes.
- Prettier: Automates code formatting to maintain a consistent style throughout your project, improving readability and maintainability.
- ESLint: Identifies and fixes errors and inconsistencies in your JavaScript code, ensuring code quality and adherence to coding standards.
- GitLens: Supercharges Git integration with features like code authorship visualization, file history exploration, and rich comparisons.
- JavaScript (ES6) code snippets: Provides pre-written code snippets for common JavaScript functionalities, saving you time and effort.
Productivity Extensions:
- GitHub Copilot: The GitHub Copilot extension in VS Code is an AI-powered code completion tool that acts like a smart assistant, suggesting code as you type to boost your development speed and accuracy.
- Code Spell Checker: Identifies and highlights potential spelling errors in your code comments, strings, and variable names.
- Settings Sync: Synchronizes your VS Code settings across different machines, ensuring a consistent development environment everywhere.
- Chrome Debugger: Enables debugging of your JavaScript code directly within Chrome from VS Code.
- IntelliCode (bundled): Provides context-aware code completions and suggestions based on your project and coding style.
- Nested Comments: Simplifies commenting and uncommenting blocks of code by allowing you to comment/uncomment entire nested sections.
React-Specific Extensions:
- Reactjs Code Snippets: Provides pre-written code snippets for common React components and functionalities, boosting your React development speed.
- ES7+ React/Redux/React-Native snippets (consider options like “ES7 React/Redux/Vue Snippets”): Offers code snippets for various elements used in React, Redux, and React-Native development.
- VSCode React Refactor: Enables refactoring features like renaming components, extracting components, and converting class components to functional components.
Node & Express Extensions:
- Node.js extension: Enhances Node.js development with features like debugging, code completion, and IntelliSense for Node.js modules.
- REST Client: Send HTTP requests and view responses directly within VS Code, simplifying API testing and debugging.
MongoDB Extension:
- MongoDB for VS Code: Connects directly to your MongoDB database, allowing you to query data, explore collections, and manage your database directly from VS Code.
CSS & Sass/SCSS Extensions:
- Tailwind CSS IntelliSense: Offers intelligent code completion and suggestions specifically for Tailwind CSS classes, accelerating your development process.
- Live Sass Compiler: Compiles your Sass code into CSS live as you save changes, providing immediate feedback on your styles.
Bonus Extensions:
- Material Icon Theme: Applies a Material Design icon theme to your VS Code interface, enhancing its visual appeal.
- Live Share: Enables real-time collaboration on VS Code projects, allowing you to co-edit and share code with others simultaneously.
- Quokka.js: Acts as a JavaScript scratchpad within VS Code, letting you run code snippets and see the results instantly without needing a browser console.
Commonly Used VS Code Keyboard Shortcuts:
Keyboard shortcuts are essential for efficient code editing. VS Code offers a built-in set of shortcuts, and many extensions provide their own. Here are some fundamental shortcuts to get you started:
- File Management & User Interface:
- Open user settings:
Ctrl/Command + ,(comma)
- Open keyboard shortcuts:
Ctrl/Command + K then Ctrl/Command + S
- Open a new tab/file:
Ctrl/Command + N
- Open a new window:
Ctrl/Command + Shift + N
- Open a recent file:
Ctrl/Command + O
- Save the current file:
Ctrl/Command + S
- Close the current tab/file:
Ctrl/Command + W
- Navigate between tabs:
Ctrl + Page Up/Down
(Windows/Linux),Option + Command + left/right arrow
(Mac) - Quick open/find file:
Ctrl/Command + P
- Open command palette:
Ctrl/Command + Shift + P (or F1 / fn+F1 on Mac)
- Toggle left sidebar (with explorer):
Ctrl/Command + B
- Toggle bottom panel:
Ctrl/Command + J
- Toggle terminal:
Control (^) + `(backtick)
- Zoom in & out:
Ctrl/Command + = (in) & Ctrl/Command + - (out)
- Zen mode (full screen, minimal UI):
Ctrl/Command + K then Z
- Split editor view:
Ctrl/Command + \
- Open user settings:
- Code Editing:
- Undo:
Ctrl/Command + Z
- Redo:
Ctrl + Y
(Windows/Linux),Command + Shift + Z
(Mac) - Copy:
Ctrl/Command + C
- Paste:
Ctrl/Command + V
- Cut:
Ctrl/Command + X
- Find (check/uncheck hamburger icon for find in selection):
Ctrl/Command + F
- Find element in command palette:
Ctrl/Command + Shift + P then @
- Replace:
Ctrl + H
(Windows/Linux),Command + Option + F
(Mac) - Comment/Uncomment lines:
Ctrl/Command + /
- Indent/Outdent lines:
Tab
/Shift + Tab
- Undo:
- Navigation:
- Go to line (type line in command palette):
Ctrl/Command + G
- Move line Up/Down:
Alt + Up/Down arrow
(Windows/Linux),Option + Up/Down arrow
(Mac) - Duplicate Line:
Ctrl + Shift + D
(Windows/Linux),Command + Option + D
(Mac) - Jump to definition (symbol):
F12 (fn + F12 on Mac)
- Jump to symbol in workspace:
Ctrl/Command + Shift + O
- Switch between open files:
Ctrl + Tab
(Windows/Linux),Command + Tab
(Mac)
- Go to line (type line in command palette):
- Selection:
- Select entire line:
Ctrl/Command + L
- Select all occurrences of current selection:
Ctrl/Command + Shift + L
- Select next occurrences of current selection:
Ctrl/Command + Shift + D
- Change name of variable/class/id everywhere : (highlight first)
F2 or fn+F2 on Mac
- Select word:
Ctrl/Command + Double-click word
- Select entire line:
Pro Tip: Explore the VS Code keyboard shortcuts documentation for a comprehensive list, and customize them to your preferences for a seamless workflow. VS Code Tips & Tricks Documentation. Additional shortcut considerations.
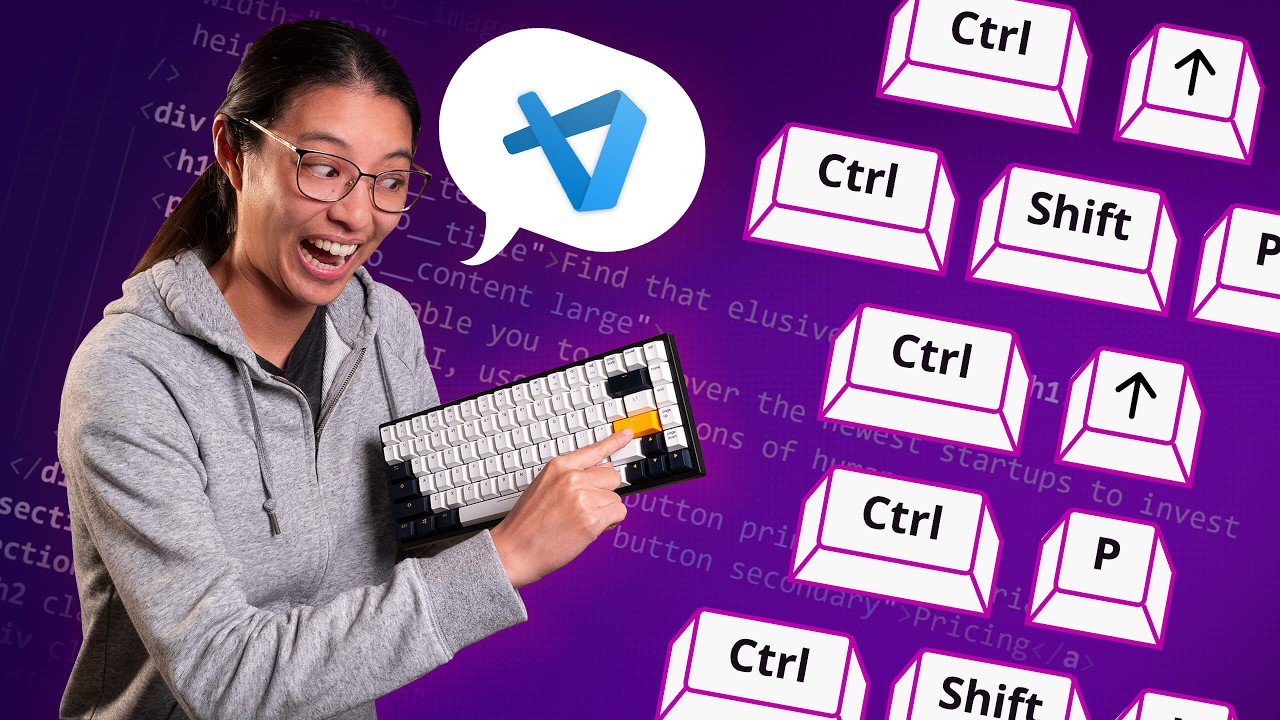
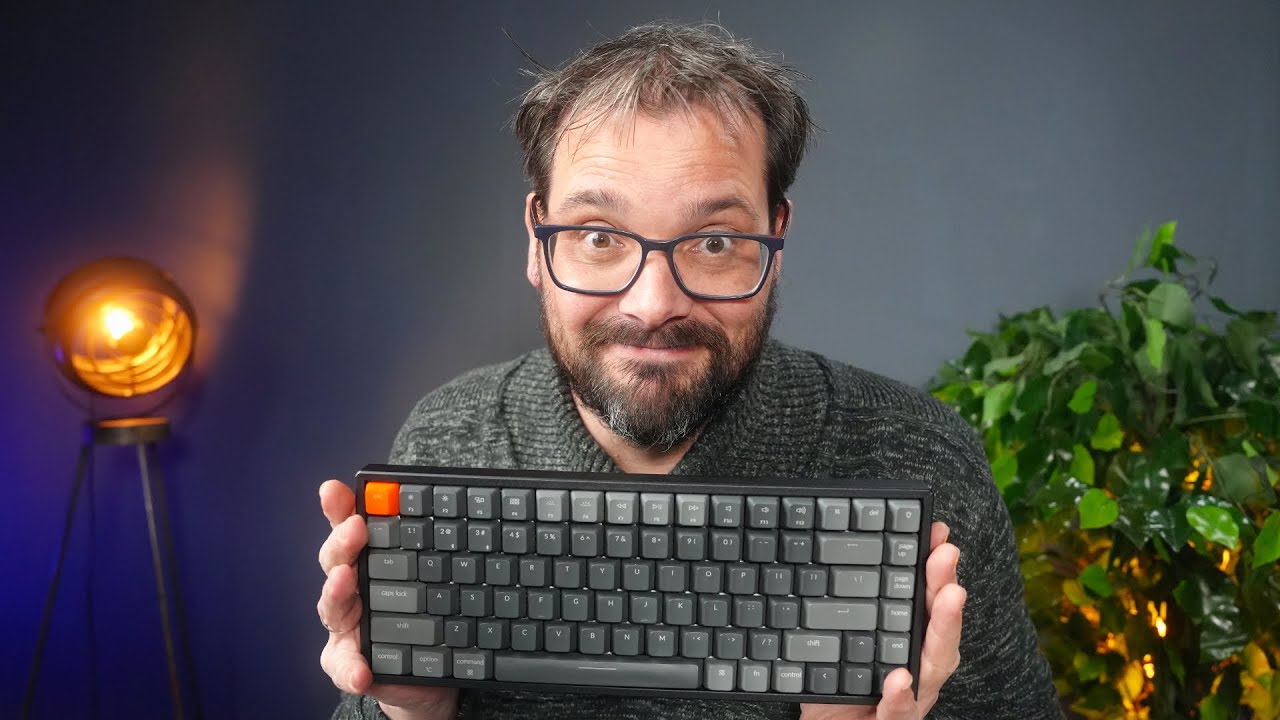
The Best Dark Mode Themes for VS Code
For many developers, dark mode themes are a must-have for reducing eye strain during long coding sessions. Here are some of the most popular dark mode themes for VS Code, offering a variety of styles to suit your taste:
- One Dark Pro: This widely used theme offers a balanced color scheme with clear syntax highlighting and a focus on readability.
- Dracula Official: Inspired by the classic Bram Stoker novel, Dracula Official boasts a deep purple base with contrasting yet pleasing colors for code elements.
- Monokai Pro: This theme provides a classic dark look with a touch of green, perfect for those who prefer a traditional feel.
- Nord: For a more subtle approach, Nord offers a cool blue color scheme that’s easy on the eyes and promotes focus.
- Cobalt2: If you enjoy a vibrant color palette, Cobalt2 delivers a dark base with pops of color for syntax highlighting, creating a visually engaging experience.
Remember: Finding the perfect theme is a personal choice. Experiment with different options and choose one that enhances your coding experience and reduces eye strain.
GitHub Copilot with VS Code: The Latest Development
GitHub Copilot is an AI-powered code completion extension that acts like an intelligent assistant for your coding projects which can be integrated with VS Code. It analyzes your code in real-time and suggests completions for:
- Functions: As you type function names, Copilot can suggest the full definition based on the context, including parameters and return types.
- Variables: Copilot can suggest variable names based on their usage and data types.
- Code Blocks: For repetitive tasks or common patterns, Copilot can generate entire code blocks, saving you time and effort.
- Documentation Comments: Copilot can even help you write documentation comments for your code, improving code readability and maintainability.
How Copilot Works with VS Code:
- Installation: You’ll need to install the GitHub Copilot extension within VS Code. This is a separate product from VS Code itself.
- Real-time Analysis: As you type code, Copilot analyzes the context, including existing code, function calls, and variables.
- Code Suggestions: Based on the analysis, Copilot suggests completions that fit seamlessly into your code. These suggestions appear in a greyed-out font alongside your code.
- Acceptance & Refinement: You can accept suggestions by pressing
Tab
or other designated shortcuts. Copilot suggestions often require some refinement, so feel free to adjust them to your specific needs. - Learning Over Time: As you use Copilot, it learns your coding style and preferences. Over time, its suggestions become more tailored to the way you code.
Benefits of Using Copilot with VS Code:
- Increased Coding Speed: Completing repetitive tasks and generating boilerplate code with Copilot saves you significant time.
- Reduced Errors: Copilot’s suggestions can help you avoid syntax errors and typos by offering correct code structures.
- Exploration of New Techniques: Copilot can expose you to alternative coding approaches or libraries you may not be familiar with.
- Improved Focus on Logic: By handling boilerplate and repetitive tasks, Copilot allows you to focus on the core logic and problem-solving aspects of your code.
Important Considerations:
- Review Suggestions: While Copilot is generally accurate, it’s crucial to review and understand its suggestions before incorporating them. Not all suggestions will be perfect, and some may require adjustments.
- Not a Replacement for Learning: Copilot is a tool to enhance your workflow, not a replacement for fundamental coding knowledge. Use it to boost your efficiency, but continue to learn and understand the underlying code principles.
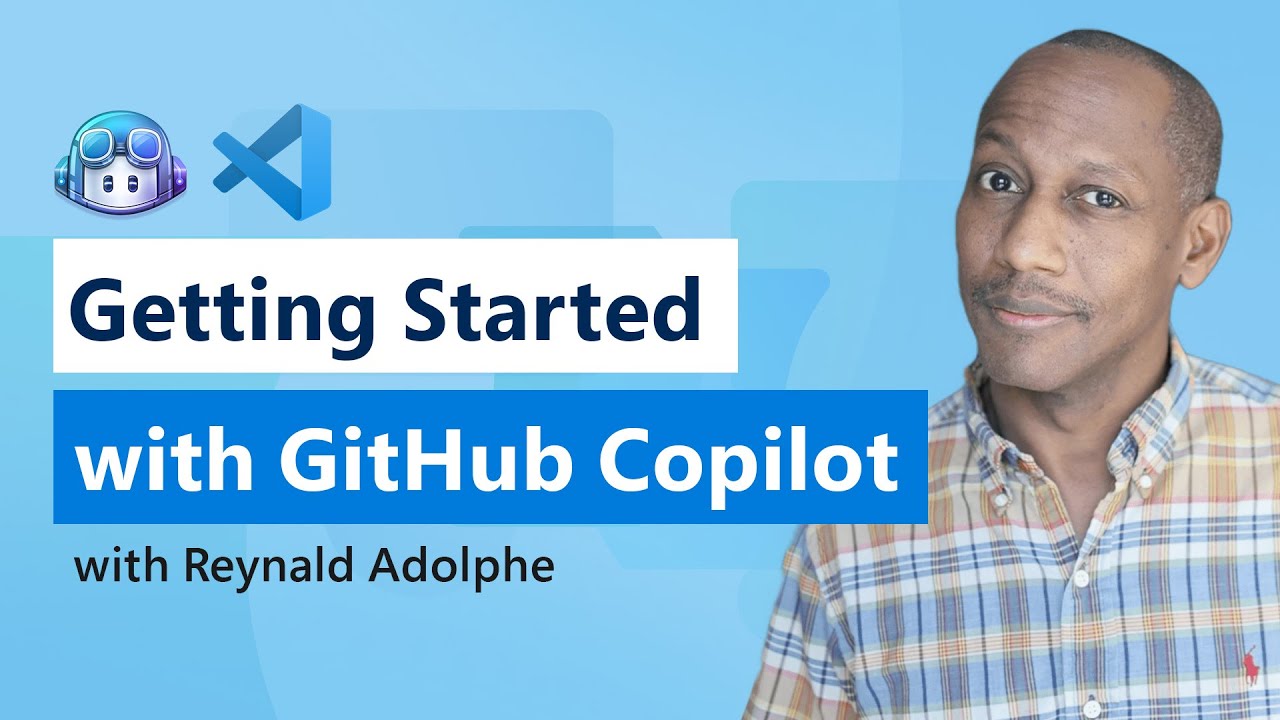
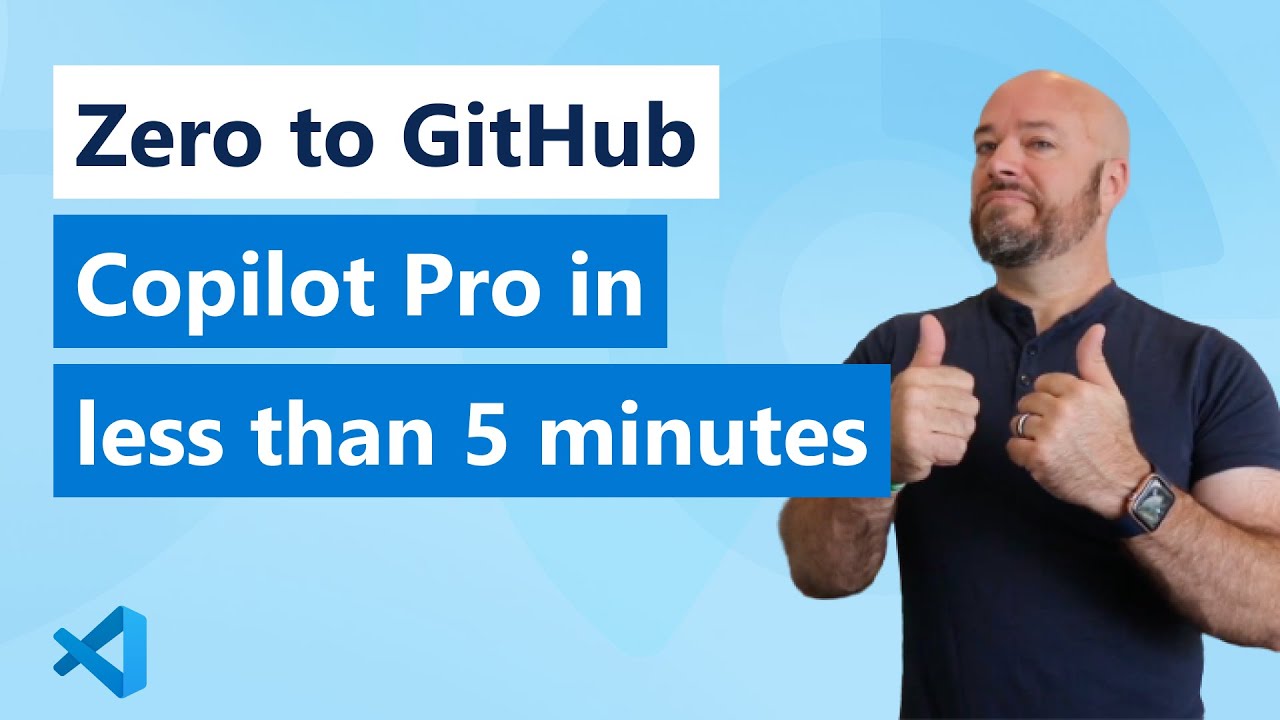
Overall, GitHub Copilot, when used effectively within VS Code, can be a game-changer for developers. It streamlines your workflow, reduces errors, and opens doors to new coding possibilities.
VS Code Complete Course Provided by FreeCodeCamp:
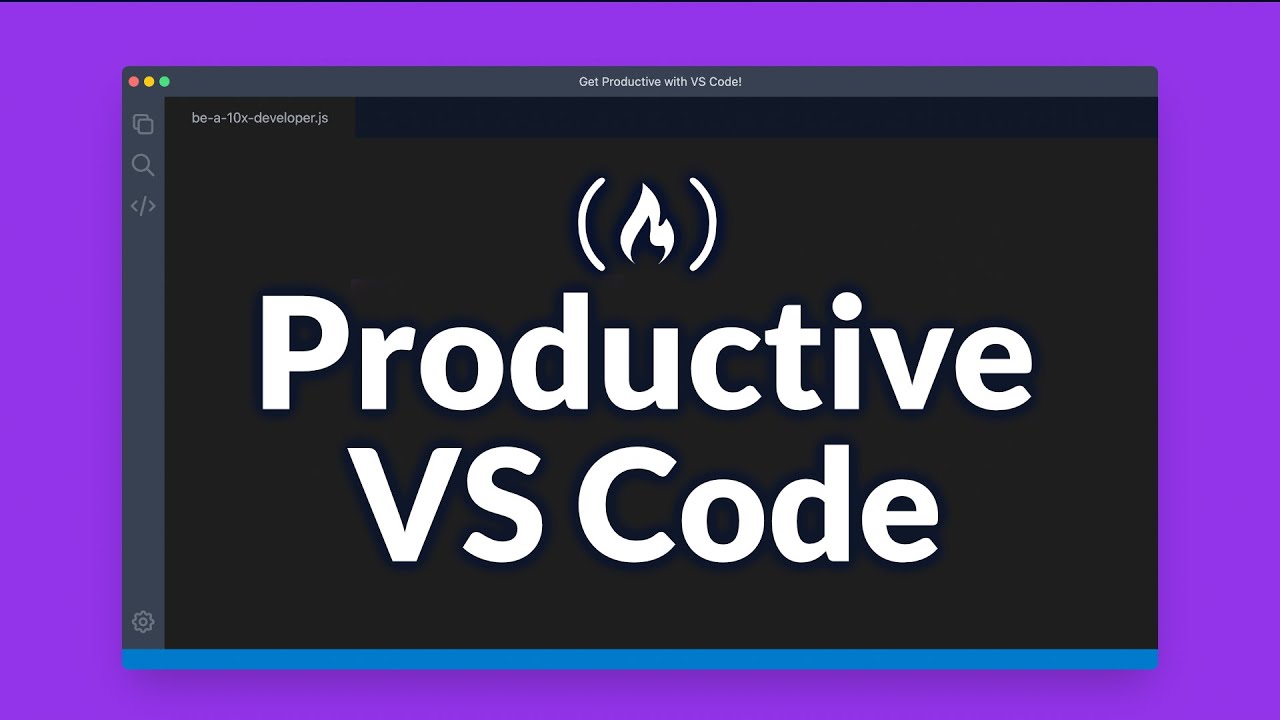